Ruby gem: distributed locking on Google Cloud
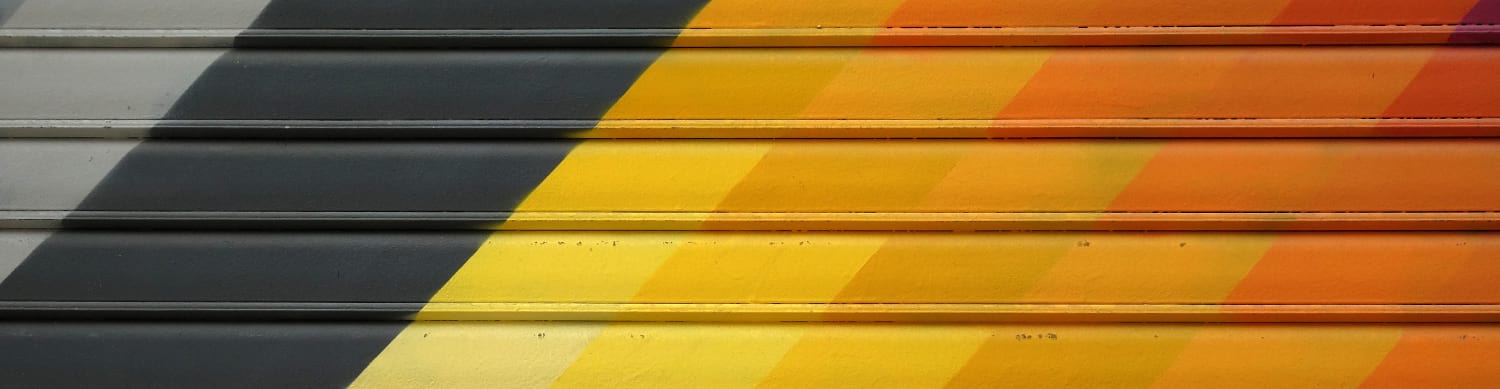
I previously designed a robust distributed locking algorithm based on Google Cloud. Now I'm releasing a Ruby implementation of this algorithm: distributed-lock-google-cloud-storage-ruby.
To use this, add to your Gemfile:
gem 'distributed-lock-google-cloud-storage'
Its typical usage is as follows. Initialize a Lock instance. It must be backed by a Google Cloud Storage bucket and object. Then do your work within a #synchronize
block.
Important: If your work is a long-running operation, then also be sure to call #check_health!
periodically to check whether the lock is still healthy. This call throws an exception if it's not healthy. Learn more in Long-running operations, lock refreshing and lock health checking.
require 'distributed-lock-google-cloud-storage'
lock = DistributedLock::GoogleCloudStorage::Lock(
bucket_name: 'your bucket name',
path: 'locks/mywork')
lock.synchronize do
do_some_work
# IMPORTANT: _periodically_ call this!
lock.check_health!
do_more_work
end
To learn more about this gem, please check out its README and its full API docs.